- CURL makes HTTP requests just like a web browser. To request a web page from the command line, type curl followed by the site's URL: The web server's response is displayed directly in your command-line interface. If you requested an HTML page, you get the page source - which is what a browser normally sees. This article covers the following.
- Curl will by default and on its own account pass a few headers in requests, like for example Host:, Accept:, User-Agent: and a few others that may depend on what the user asks curl to do. All headers set by curl itself can be overridden, replaced if you will, by the user.
- Curl lets you quickly download files from a remote system. Curl supports many different protocols and can also make more complex web requests, including interacting with remote APIs to send and receive data. You can learn more by viewing the manual page for curl by running man curl.
These curl recipes show you how to send POST requests with curl. By default, curl sends GET requests. To make it send POST requests, use the -X POST
command line argument. To add POST data to the request, use the -d
argument. To change the Content-Type
header that tells the web server what type of data you're sending, use the -H
argument.
Make a request from Curl using mutual TLS Now, we need only to configure our Curl client to make authenticated requests using our certificate and private key. The CA root certificate will be used to verify that the client can trust the certificate presented by the server.
Send an Empty POST Request
This recipe uses the -X POST
option that makes curl send a POST request to https://catonmat.net
. Once it receives a response from the server, it prints the response body to the screen. It doesn't send any POST data.
Send a POST Request with Form Data
This recipe sends a POST request to https://google.com/login
with login=emma&password=123
data in request's body. When using the -d
argument, curl also sets the Content-Type
header to application/x-www-form-urlencoded
. Additionally, when the -d
option is set, the -X POST
argument can be skipped as curl will automatically set the request's type to POST.
Skipping the -X Argument
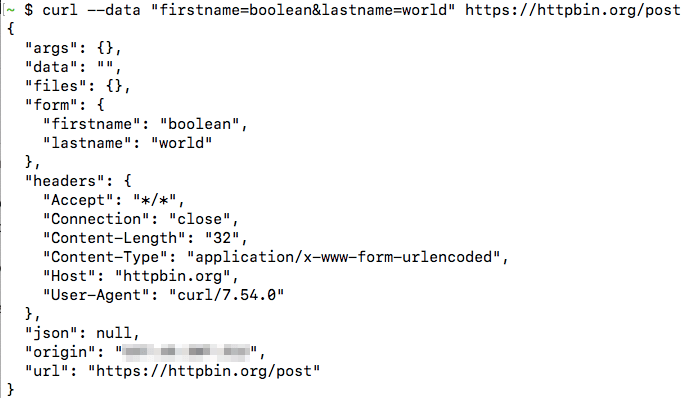
In this recipe, we skip the -X POST
argument that explicitly tells curl to send a POST request. We can skip it because we have specified the -d
argument. When -d
is used, curl implicitly sets the request's type to POST.
A Neater Way to POST Data
The previous recipe used a single -d
option to send all data login=emma&password=123
but here's a neater way to do the same that makes the command easier to read. Instead of using a single -d
argument for all data, use multiple -d
arguments for each key=value
data piece! If you do that, then curl joins all data pieces with the &
separator symbol when creating the request. This recipe skips the -X POST
argument because when -d
argument is present, curl makes a POST request implicitly.
Send a POST Request and Follow a Redirect
This recipe uses the -L
command line option that tells curl to follow any possible redirects that it may encounter in the way. By default, curl doesn't follow the redirects, so you have to add -L
to make it follow them. This recipe skips the -X POST
argument because -d
argument forces curl to make a POST request.
Send a POST Request with JSON Data
In this recipe, curl sends a POST request with JSON data in it. This is accomplished by passing JSON to the -d
option and also using the -H
option that sets the Content-Type
header to application/json
. Setting the content type header to application/json
is necessary because otherwise, the web server won't know what type of data this is. I've also removed the -X POST
argument as it can be skipped because -d
forces a POST request.
Send a POST Request with XML Data
This recipe POSTs XML data to https://google.com/login
. Just like the form data and JSON data, XML data is specified in the -d
argument. To tell the web server that the request contains XML, the Content-Type
header is changed to text/xml
via the -H
argument.
Send a POST Request with Plain Text Data
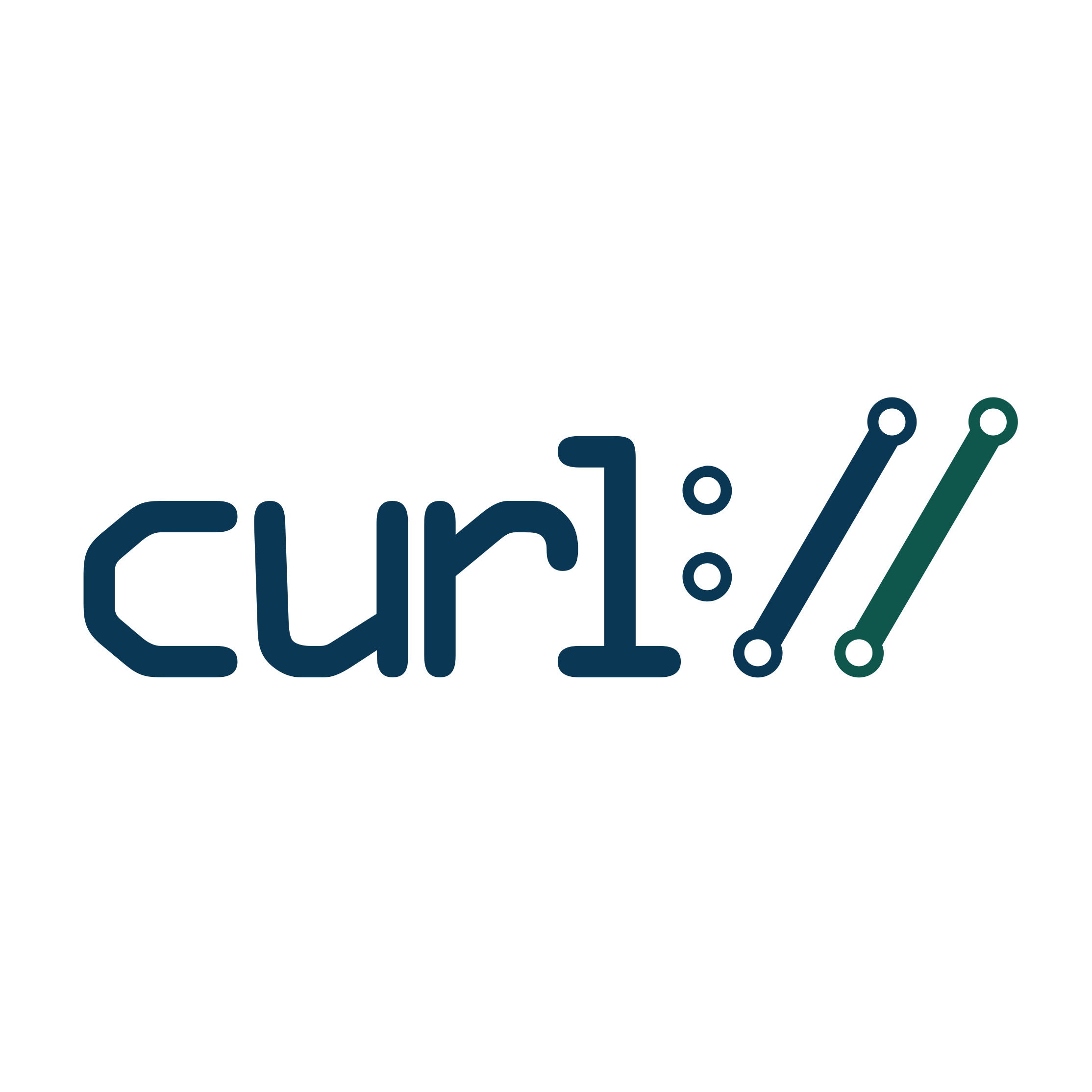
This recipe sends a POST request with a plain text string hello world
in request's body. It also sets the Content-Type
header to text/plain
to tell web server that it's just plain text coming in.
Send a POST Request with Data from a File
This recipe loads POST data from a file called data.txt
. Notice the extra @
symbol before the filename. That's how you tell curl that data.txt
is a file and not just a string that should go in the POST body.
URL-encode POST Data
So far, all recipes have been using the -d
argument to add POST data to requests. This argument assumes that your data is URL-encoded already. If it is not, then there can be some trouble. If your data is not URL-encoded, then replace -d
with --data-urlencode
. It works exactly the same way as -d
, except the data gets URL-encoded by curl before it's sent out on the wire.
POST a Binary File
This recipe uses the -F
argument that forces curl to make a multipart form data POST request. It's a more complicated content type that's more efficient at sending binary files. This recipe makes curl read an image photo.png
and upload it to https://google.com/profile
with a name file
. The -F
argument also sets the Content-Type
header to multipart/form-data
.
Python Curl To Requests
POST a Binary File and Set Its MIME Type
Similar to the previous recipe, this recipe uses the -F
argument to upload a binary file (a photo with the filename photo.png
) via a multipart POST request. It also specifies the MIME type of this file and sets it to image/png
. If no type is specified, then curl sets it to application/octet-stream
.
POST a Binary File and Change Its Filename
Similar to the previous two recipes, this recipe uses the -F
argument to upload a photo.png
via a POST request. Additionally, in this recipe, the filename that is sent to the web server is changed from photo.png
to me.png
. The web server only sees the filename me.png
and doesn't know the original filename was photo.png
.
Created by Browserling
These curl recipes were written down by me and my team at Browserling. We use recipes like this every day to get things done and improve our product. Browserling itself is an online cross-browser testing service powered by alien technology. Check it out!
Secret message: If you love my curl recipe, then I love you, too! Use coupon code CURLLING to get a discount at my company.
This post is part of a wider series on CURL, a very useful tools utilised extensively either straight from the command line, or through scripting language specific variants.
GET is one of the most commonly used HTTP methods, and is used to request data from a specified URL.
Curl Requests Per Second
When making a GET request, remember that the query string (as in, the key/value pairs) make up the URI of the request itself:
Also, remember:
- GET requests can be cached
- GET requests remain in the browser history
- GET requests can be bookmarked
- GET requests should never be used when dealing with sensitive data
- GET requests have length restrictions
- GET requests is only used to request data (not modify)
With that in mind, let’s see how it’s done and run through a couple of ways to deal with the result too.
Perform a GET Request with CURL on the Command Line
Performing a GET request is simple with CURL, simply have CURL hit the URL you wish with no additional flags or parameters:
Here you can see I sent a GET request to httpbin.org, and got a response telling me a bit about myself such as my User-Agent, IP, etc…
Save CURL GET Request Response to File on the Command Line
Perhaps you want to save the contents of the servers response to a file for use, you can easily do this using CURL’s “-o” flag like this:
I called the file “response.json” because I knew the server was going to deliver a JSON response back to me, if you’re expecting an XML response, or something else, then name your file appropriately.
I can now open the file and see it’s content like so:
Retrieve Only Response Code of a GET Request with CURL on the Command Line
If you aren’t interested in the contents of the GET request, though only want to see the response code (to see if the endpoint is up and working for example), you can use the following:
Curl To Requests Python
A 200 response indicates the request was successful, whereas a 404 means the endpoint hasn’t been found, or a 500 would suggest there’s an issue at the servers side.
Curl Requests To Json
Be sure to read through the other posts on CURL in this series to get to grips with this powerful tool.
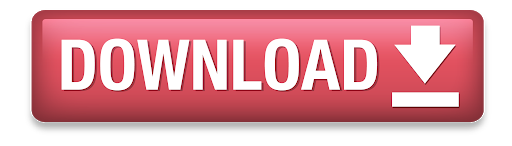